OOP: Understanding Object-Oriented Programming in Python
Today, I would like to talk about Object-Oriented Programming (OOP) in Python. It’s not a secret that the very concept of OOP for some beginner programmers (I had such acquaintances) is causing perplexity and questions: “Why do I need OOP? I’m already good at writing programs, processing various data, creating conditions, loops, functions, and writing […]
Technologies
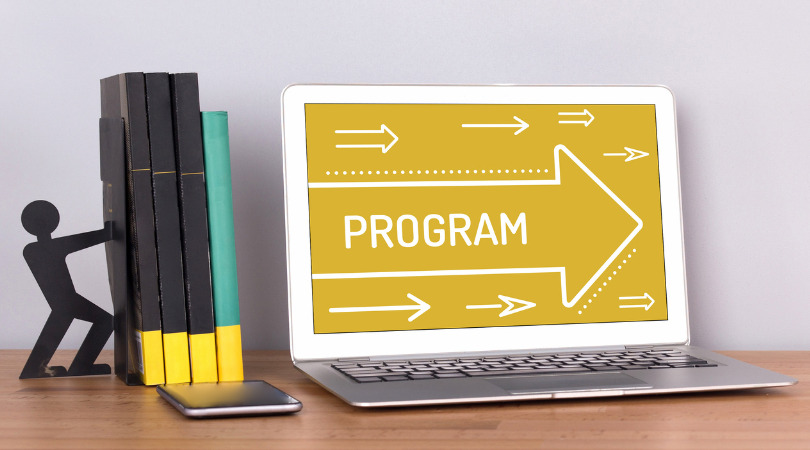
Today, I would like to talk about Object-Oriented Programming (OOP) in Python.
It’s not a secret that the very concept of OOP for some beginner programmers (I had such acquaintances) is causing perplexity and questions: “Why do I need OOP? I’m already good at writing programs, processing various data, creating conditions, loops, functions, and writing programs of almost any complexity.”
Yes, I agree, this was the case until 1990 after which the concept of OOP became the dominant direction. In the next 10-15 years, it was actively introduced into the most popular programming languages. And nowadays, I believe that every beginner programmer should know OOP because we almost always represent everything as data objects in our programs.
For example, when creating the program for selling tickets to the cinema we are dealing with “Tickets” objects.
Ticket Example
The “Tickets” object itself can have such properties as:
- Movie title
- Date and time of the visit
- Hall number
- Row number
- Place number
Thanks to the existence of the objects, it will be very convenient to bring all this ticket-related data into one place.
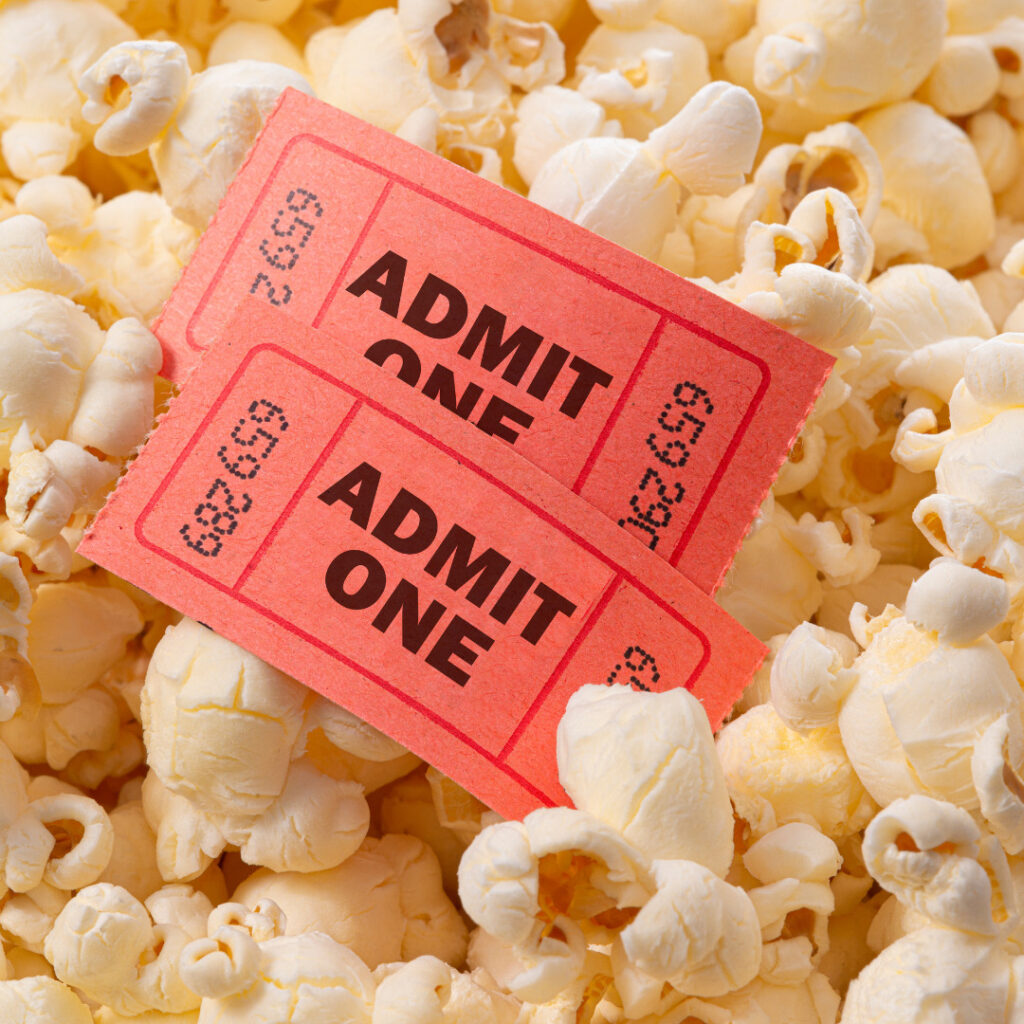
A class is created with the name “Tickets” and this class can be perceived as a kind of template. Later, objects with the ticket data will be created on it. Let’s add all the above properties to this class: movie title, date, time, etc. You can define the set of these properties yourself.
After creating the class (so to speak, template) you can create tickets with data, they will be called “Objects” or “Class Instances,” as they are created in the image and likeness of the “Tickets” class. These objects will already contain specific data about the movie title, the date and time of the show, the hall, the row, and the specific place purchased.
Further, we’ll be able to work with each ticket created on the basis of the class as a separate object. This is very convenient.
And so, at this stage, we already understand what the class (so-called template for the future class instances) and the class instances (creation based on a template) are:
The class can also contain methods (sets of functions) for working both with the class and with its objects. For example, the method that will change the ticket status to “Used” so that multiple people cannot enter the hall with one ticket. Accordingly, this status change method can be called separately for each object, and it will work for each object independently of other objects.
Encapsulation
Also, the class may have some internal methods for “hidden” manipulation. In this case, they are inside the class and practically inaccessible from the outside. Other programmers working with this class cannot access hidden methods.
In our example, when we buy a movie ticket only “Public Information” is important to us – for which movie the ticket was bought, date, time, hall, row, and place. But we absolutely do not care about the name of the seller who has sold it to us, whether the ticket was bought by going through the referral program or it was a direct transition to the site and other internal logic for analytics.
That is, imagine that one Ticket as a whole is the kind of “Capsule” that hides the huge amount of various statistical data, data for analytics, accounting, and other information inside. The internal content of the Ticket is not absolutely important to the ordinary buyer except for its public information – five fields, so the rest of the ticket is hidden and made available only from the “inside of the ticket”.
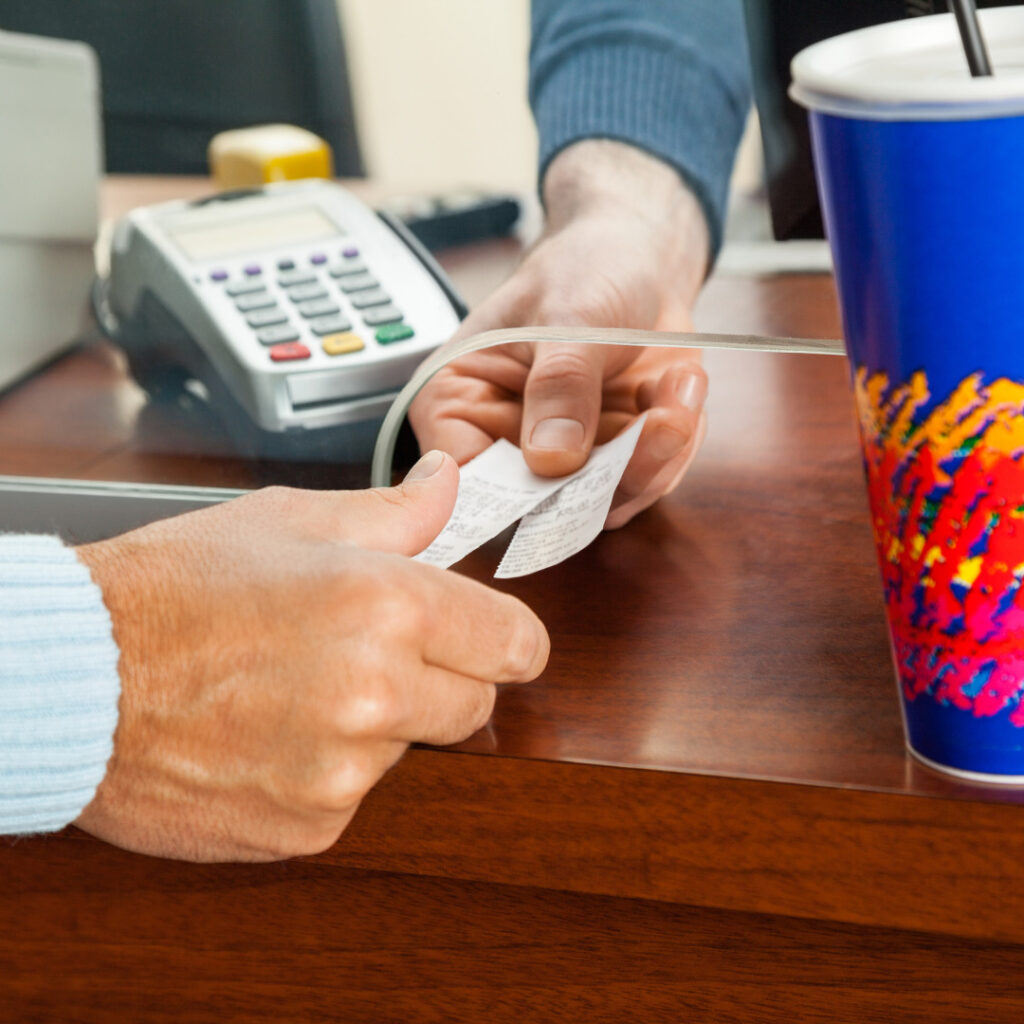
This hiding mechanism is called Encapsulation and is indicated at the beginning of the property or method name:
Protected access level, indicated by a single “_” underscore
Private access level, indicated by a double “__” underscore
And then, encapsulation is when the hidden data and methods cannot be accessed from the outside, only inside the class.
Inheritance
Now let’s imagine that the owner of the cinema chain has decided to open a zoo. Now, he needs to sell tickets to the zoo as well.
The main part of the zoo ticket’s functionality is similar to the ticket to the cinema, with the exception of some public data. For example, we do not need the name of the movie, the data of the hall, row, or place anymore.
In order not to create a new class for tickets to the Zoo and duplicate huge parts of the code – we can use the OOP mechanism known as Inheritance.
To do this, we just need to create the separate, abstract base class “Tickets,” leave only common properties and methods for all types of tickets in it, and then inherit from it in the right places.
That is, for the tickets to the Cinema, we inherit from the base class “Tickets” and add four missing properties to it: the movie title, hall, row, and place.
For tickets to the Zoo, we simply inherit from the “Tickets” base class and do not add anything else to it because here we have enough basic properties and methods.
Polymorphism
This OOP concept has two definitions. The first one means that we can work with different data types in a unified way. The second one describes the possibility of processing completely different objects by using the same method name.
I will try to describe this with the simple example of buying tickets next.
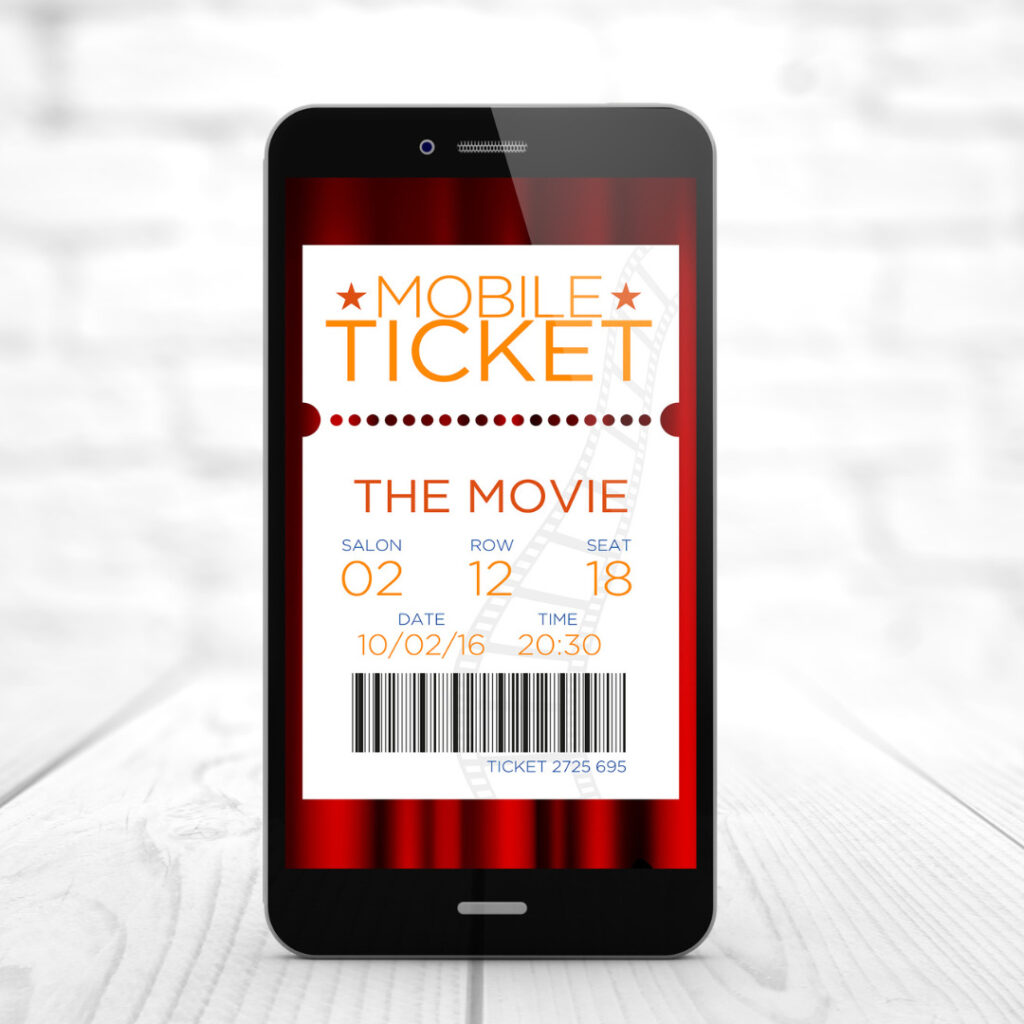
The cinema’s hall name can be either just a number or a verbal name like “CINETECH,” “RE’LUX,” or “IMAX”. So, when creating the class, the type INT was immediately set for the hall number. Later, when creating the object and sending the name “IMAX” instead of the hall number to it, the program will generate an error. To avoid this, the concept of Polymorphism is applied, thanks to which, additional processing checks are put inside the methods.
Conclusion
Such, briefly, and I hope that it was clear, describe the principles of Object-Oriented Programming in Python. Thank you for having read this far. I will write my next article about Generators and Iterators in Python.
If you need a custom and high-quality solution, contact Swan Software Solutions for a free assessment.